Frontend SEO Best Practices - Essential Techniques for Developers
Share this post
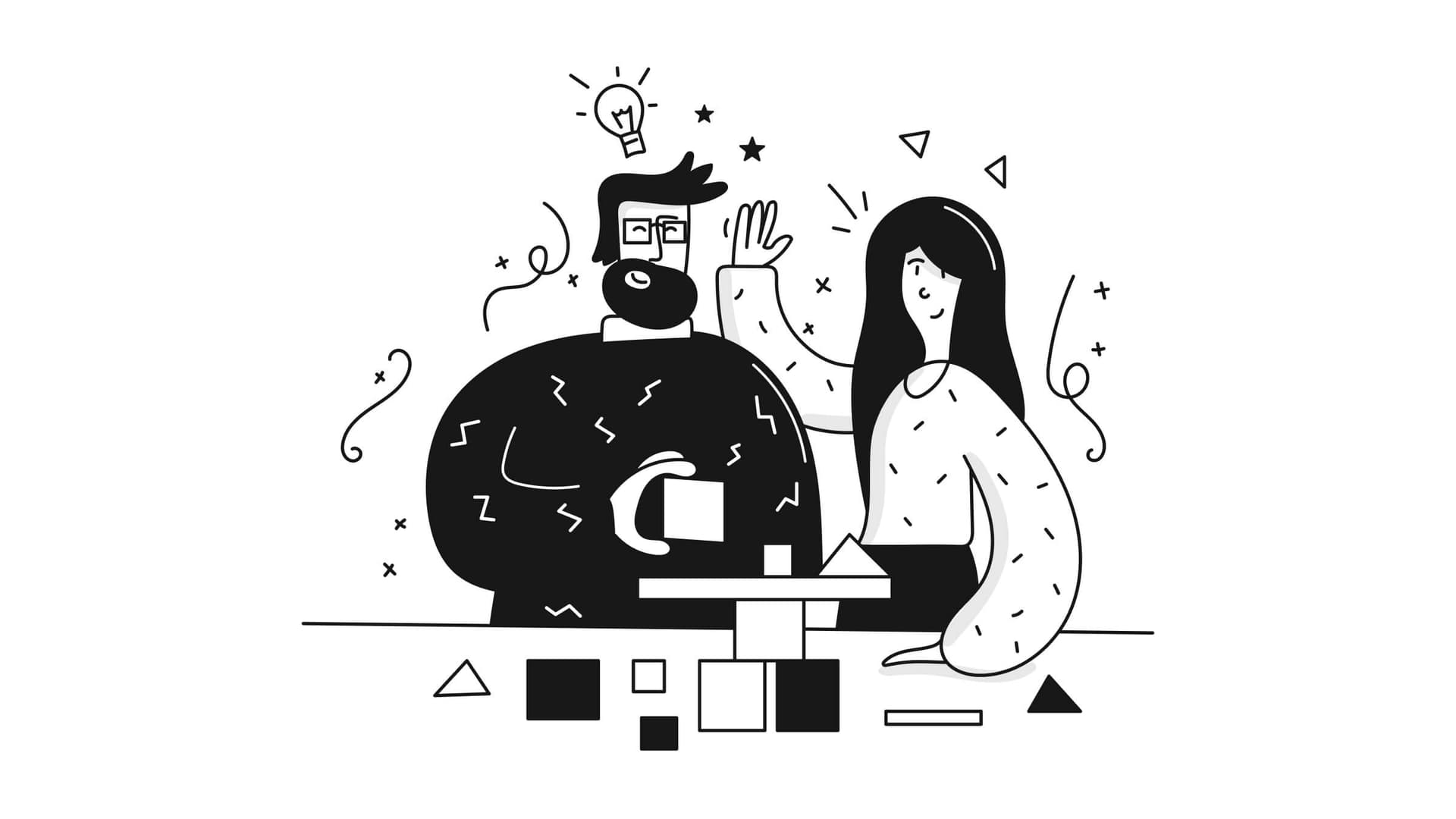
Frontend SEO Best Practices: Essential Techniques for Developers
Frontend developers play a crucial role in ensuring websites are optimized for search engines. While backend architecture and content are important, how you structure and implement your frontend code can significantly impact a site's visibility and rankings.
Semantic HTML: The Foundation of SEO-Friendly Code
Using semantic HTML elements appropriately is one of the most fundamental practices for SEO:
<!-- Poor for SEO -->
<div class="header">
<div class="nav">Menu items</div>
</div>
<div class="main-content">
<div class="title">Page Title</div>
<div class="content">Content here...</div>
</div>
<!-- Better for SEO -->
<header>
<nav>Menu items</nav>
</header>
<main>
<h1>Page Title</h1>
<article>Content here...</article>
</main>
Semantic elements like <header>
, <nav>
, <main>
, <article>
, <section>
, <aside>
, and <footer>
provide context about your content's role and importance, helping search engines better understand your page structure.
Optimizing Page Speed Through Frontend Code
Page speed is a direct ranking factor for both mobile and desktop searches. Frontend developers can implement several techniques to improve load times:
1. Efficient CSS Architecture
/* Avoid large CSS files with unused styles */
/* Instead, consider CSS-in-JS or utility-first approaches */
/* For critical CSS, inline in the head */
<head>
<style>
/* Critical CSS here */
</style>
<link rel="stylesheet" href="/non-critical.css" media="print" onload="this.media='all'">
</head>
2. JavaScript Optimization
// Use code splitting in modern frameworks
import { Suspense, lazy } from 'react'
const HeavyComponent = lazy(() => import('./HeavyComponent'))
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<HeavyComponent />
</Suspense>
)
}
3. Image Optimization
Modern image formats and lazy loading are essential:
<!-- Responsive images with WebP support -->
<picture>
<source srcset="image.webp" type="image/webp" />
<source srcset="image.jpg" type="image/jpeg" />
<img
src="image.jpg"
alt="Descriptive alt text"
loading="lazy"
width="800"
height="600"
/>
</picture>
Implementing Structured Data
Structured data helps search engines understand your content and can lead to rich snippets in search results:
<script type="application/ld+json">
{
"@context": "https://schema.org",
"@type": "Article",
"headline": "Frontend SEO Best Practices",
"image": "https://example.com/article-image.jpg",
"author": {
"@type": "Person",
"name": "Michael Chen"
},
"publisher": {
"@type": "Organization",
"name": "StartSEO UP",
"logo": {
"@type": "ImageObject",
"url": "https://example.com/logo.png"
}
},
"datePublished": "2025-05-30",
"dateModified": "2025-05-30"
}
</script>
Mobile-First Development for SEO
Google's mobile-first indexing means your mobile experience is prioritized in rankings:
/* Start with mobile styles as the default */
.container {
padding: 1rem;
width: 100%;
}
/* Then add desktop styles with media queries */
@media (min-width: 768px) {
.container {
padding: 2rem;
max-width: 1200px;
margin: 0 auto;
}
}
Managing JavaScript for Crawlability
JavaScript frameworks can create crawling challenges for search engines:
Server-Side Rendering (SSR)
// Next.js example of SSR
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/data')
const data = await res.json()
return {
props: { data },
}
}
Static Site Generation (SSG)
// Next.js example of SSG
export async function getStaticProps() {
const res = await fetch('https://api.example.com/data')
const data = await res.json()
return {
props: { data },
revalidate: 60, // Regenerate page after 60 seconds
}
}
Accessible Frontend Code Improves SEO
Accessibility and SEO are closely aligned - both aim to make content more understandable:
<!-- Add proper ARIA attributes -->
<button
aria-expanded="false"
aria-controls="dropdown-menu"
aria-label="Open menu"
>
Menu
</button>
<div id="dropdown-menu" hidden>
<!-- Menu items -->
</div>
Implementing Canonical URLs
Prevent duplicate content issues with proper canonical tags:
<!-- In the head section -->
<link rel="canonical" href="https://example.com/original-page" />
Conclusion
Frontend development is no longer just about creating beautiful interfaces. As search engines become more sophisticated in how they evaluate websites, the technical implementation details have a direct impact on SEO performance. By incorporating these frontend SEO practices into your development workflow, you'll create websites that are not only user-friendly but also optimized for search engine visibility.
Remember that frontend SEO is an ongoing process. Stay updated with search engine algorithm changes and continually refine your approach to maintain and improve your rankings over time.