Building SEO-Friendly React Applications - A Complete Guide
Share this post
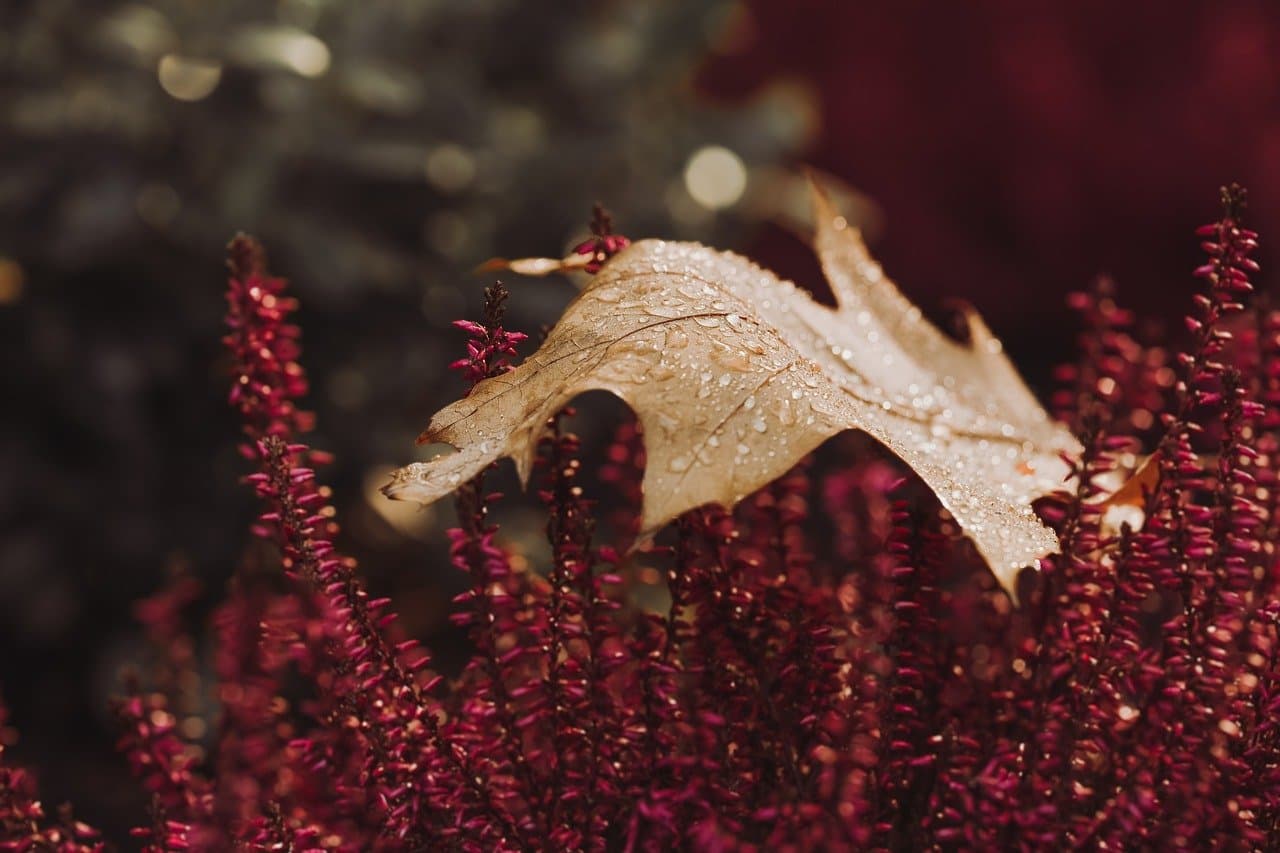
Building SEO-Friendly React Applications: A Complete Guide
React has revolutionized frontend development with its component-based architecture and virtual DOM. However, single-page applications (SPAs) built with React often face SEO challenges due to their client-side rendering nature. In this guide, we'll explore practical strategies to create React applications that perform well in search engines.
The SEO Challenges with React Applications
Traditional React applications have several limitations when it comes to SEO:
- Client-side rendering - Content isn't available during the initial HTML response
- Delayed content loading - Search engine crawlers may not wait for JavaScript execution
- Dynamic routing - URL changes managed by JavaScript can be difficult for crawlers to discover
- Performance issues - Large bundle sizes can lead to slow page loads
Server-Side Rendering (SSR) with React
Server-side rendering generates HTML on the server before sending it to the client, making content immediately available to search engines.
Next.js: The React Framework with Built-in SSR
Next.js has become the go-to solution for SEO-friendly React applications:
// pages/blog/[slug].js
export async function getServerSideProps({ params }) {
// Fetch data from an API or database
const res = await fetch(`https://api.example.com/posts/${params.slug}`)
const post = await res.json()
return {
props: { post }, // Will be passed to the page component as props
}
}
function BlogPost({ post }) {
return (
<article>
<h1>{post.title}</h1>
<div dangerouslySetInnerHTML={{ __html: post.content }} />
</article>
)
}
export default BlogPost
Static Site Generation (SSG) for Even Better Performance
For content that doesn't change frequently, static generation provides the best performance:
// pages/blog/[slug].js
export async function getStaticPaths() {
// Fetch all possible blog post slugs
const res = await fetch('https://api.example.com/posts')
const posts = await res.json()
const paths = posts.map((post) => ({
params: { slug: post.slug },
}))
return { paths, fallback: 'blocking' }
}
export async function getStaticProps({ params }) {
// Fetch data for a specific blog post
const res = await fetch(`https://api.example.com/posts/${params.slug}`)
const post = await res.json()
return {
props: { post },
revalidate: 3600, // Re-generate page at most once per hour
}
}
function BlogPost({ post }) {
// Same component as above
}
export default BlogPost
React Helmet for Managing Document Head
For traditional React applications, React Helmet allows you to manage the document head:
import { Helmet } from 'react-helmet'
function ProductPage({ product }) {
return (
<div>
<Helmet>
<title>{product.name} | StartSEO UP</title>
<meta name="description" content={product.description} />
<link
rel="canonical"
href={`https://startseo-up.com/products/${product.slug}`}
/>
<meta property="og:title" content={product.name} />
<meta property="og:description" content={product.description} />
<meta property="og:image" content={product.image} />
</Helmet>
{/* Product content */}
</div>
)
}
Code Splitting for Performance
Large React applications can lead to slow initial load times. Code splitting helps by breaking your code into smaller chunks:
import React, { Suspense, lazy } from 'react'
import { Route, BrowserRouter as Router, Switch } from 'react-router-dom'
// Dynamic imports for code splitting
const Home = lazy(() => import('./pages/Home'))
const About = lazy(() => import('./pages/About'))
const Products = lazy(() => import('./pages/Products'))
const Contact = lazy(() => import('./pages/Contact'))
function App() {
return (
<Router>
<Suspense fallback={<div>Loading...</div>}>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/products" component={Products} />
<Route path="/contact" component={Contact} />
</Switch>
</Suspense>
</Router>
)
}
Using Prerendering for Simple SPAs
For smaller React applications without server capabilities, prerendering can be a solution:
# Using react-snap in your package.json scripts
"postbuild": "react-snap"
Configuration in your package.json
:
"reactSnap": {
"include": [
"/",
"/about",
"/products",
"/contact"
],
"skipThirdPartyRequests": true
}
Implementing Structured Data in React
Adding structured data helps search engines understand your content better:
function ProductSchema({ product }) {
const schema = {
'@context': 'https://schema.org',
'@type': 'Product',
name: product.name,
image: product.image,
description: product.description,
brand: {
'@type': 'Brand',
name: 'StartSEO UP',
},
offers: {
'@type': 'Offer',
price: product.price,
priceCurrency: 'USD',
availability: product.inStock ? 'InStock' : 'OutOfStock',
},
}
return (
<script
type="application/ld+json"
dangerouslySetInnerHTML={{ __html: JSON.stringify(schema) }}
/>
)
}
Optimizing React Performance for Better SEO
Performance is a direct ranking factor for SEO. Here are some React-specific optimizations:
Component Memoization
import React, { memo } from 'react'
const ExpensiveComponent = memo(function ExpensiveComponent({ data }) {
// Complex rendering logic
return <div>{/* Rendered content */}</div>
})
Using the Production Build
Always ensure you're using the production build for deployed applications:
// webpack.config.js
module.exports = {
mode: 'production',
// Other configurations
}
Implementing Dynamic Sitemaps
Generate dynamic sitemaps for your React application:
// pages/sitemap.xml.js (Next.js)
import { getAllPosts, getAllProducts } from '../lib/api'
const Sitemap = () => {
// Component returns null as this is an XML response
return null
}
export async function getServerSideProps({ res }) {
const posts = await getAllPosts()
const products = await getAllProducts()
const baseUrl = 'https://startseo-up.com'
const sitemap = `<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
<url>
<loc>${baseUrl}</loc>
<lastmod>${new Date().toISOString()}</lastmod>
<changefreq>daily</changefreq>
<priority>1.0</priority>
</url>
${posts
.map((post) => {
return `
<url>
<loc>${baseUrl}/blog/${post.slug}</loc>
<lastmod>${post.updatedAt}</lastmod>
<changefreq>weekly</changefreq>
<priority>0.8</priority>
</url>
`
})
.join('')}
${products
.map((product) => {
return `
<url>
<loc>${baseUrl}/products/${product.slug}</loc>
<lastmod>${product.updatedAt}</lastmod>
<changefreq>weekly</changefreq>
<priority>0.8</priority>
</url>
`
})
.join('')}
</urlset>
`
res.setHeader('Content-Type', 'text/xml')
res.write(sitemap)
res.end()
return {
props: {},
}
}
export default Sitemap
Conclusion
Building SEO-friendly React applications requires thoughtful architecture decisions. By leveraging server-side rendering, optimizing performance, and implementing proper metadata, you can create React applications that rank well in search engines.
The choice between Next.js (or similar frameworks) and traditional React SPAs should be influenced by your SEO requirements. For content-heavy sites where search visibility is crucial, server rendering approaches are strongly recommended. For applications where search traffic is less important, client-side rendering with some SEO enhancements may be sufficient.